Introduction
In this article you will find out how to use the webcam integrated on Raspberry: the PiCamera. This additional module is an accessory to be reckoned with and I highly recommend to you if you wish to explore the world of Raspberry: the ability to take pictures and videos in HD. But also to make image analysis in Python, thanks to the libraries that makes it possibible, including OpenCV.
This is an introductory article of a series of articles that will cover gradually more and more detail, the fascinating world of images analysis. You will do this using the Python language.
The PiCamera v2
Released in April 2016, the PiCamera v2 is the new version of this accessory, which went to replace the now old model PiCamera v1.3. It remains compatible with all existing Raspberry models. In fact, the flat cable has remained unchanged, still suitable to be inserted into the dedicated connector on the upper side of all the Raspberry boards.
This new module mounts a Sony IMX219 camera with 8-megapixel camera, so replacing the old OmniVision OC5647 with 5-megapixel on the previous model.
Installing the PiCamera
As I wrote before, the PiCamera is a module designed to be used on Raspberry, and therefore has a dedicated port on the card. So with the Pi off (I recommend), insert the flat cable, making sure that the blue side faces the Ethernet port. After connecting the WebCam turn on the Raspberry Pi.
Once the Raspbian system is activated, launch a terminal session and enter the following command to the configuration.
$ sudo raspi-config
In the displaying menu, select Enable Camera, and then select Enable. Select Finish and finally press Yes to reboot.
Wait for the reboot of the Raspbian. Now you have to test if the PiCamera works fine. Open again a command console, and enter the following command:
raspistill -o image.jpg
On the screen you should see a preview of a few seconds and then a new file with the captured image will be saved on the current directory.
Using the PiCamera with Python
There is a module in Python to use the PiCamera with the Python language, its name is python-picamera. This module was developed by Dave Jones ( see here). With this library you can write programs that allow you to take pictures, make videos and then process them later. Also thanks to the GPIO pins it will be possible to integrate the camera using sensors or switches.
Thus, to get start with Python and the PiCamera you have to install the necessary modules:
$ sudo apt-get install python-picamera python3-picamera python-rpi.gpio
Once all the modules installed, you’re ready to test your PiCamera with the Python language. Create a new file and save it as get_image.py (Do not save it as picamera.py ๐ )
$ nano get_image.py
First, you will need to import the PiCamera class, it will generally correspond to your WebCam on which your commands directly refer. Import also the time module. You need later for managing the waiting times between a command and the next.
from picamera import PiCamera import time
Now you can enter commands to the acquisition of an image that will be saved as JPG files directly on the file system.
camera = PiCamera() camera.start_preview() time.sleep(10) camera.capture('/home/pi/image.jpg') camera.stop_preview()
Save the code pressing CTRL+O and the exit with CTRL+X. Now you can execute it
$ python get_image
You should see the preview from the WebCam. It will remain active for 10 seconds, takes a picture, before it closes. The delay is necessary to allow the sensor to set the levels of light before taking the picture.
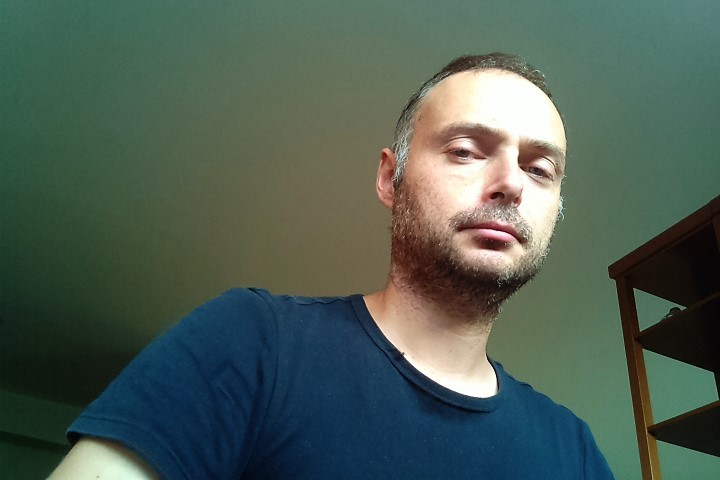
If during the preview you’ll have to get a picture reversed, ie upside down, there is no need to reverse the PiCamera, but enter the command in the code, just before the calling to the start_preview() function.
camera.rotation = 180
Now, after you checked that the PiCamera is working properly, and find out how you can use it with Python, it is time for you to try some simple tasks that you will find helpful to improve your approach to this WebCam.
Taking a sequence of pictures
Starting from the example above, the modificherai in order to obtain a sequence of images. For example, if you want to take a sequence of nine photos, you will need to write the previous code into a loop.
from picamera import PiCamera import time camera.start_preview() for i in range(9): time.sleep(5) camera.capture('/home/pi/image%s.jpg' % i) camera.stop_preview()
Looking at the above script, it’s easy to see how you can add a number to the end of the file name, to avoid that the JPG file is not overwritten.
Now it would be interesting to take all these photos and make a movie! For this purpose you can use avconv. If this application is not installed on your Raspbian, you can do it entering the following commands:
$ sudo apt-get install libavcodec-extra $ sudo apt-get install libav-tools
Once you install the codec and avconv (within libav-tools). You can create the video combining the sequence of images (stop-motion videos).
$ avconv -r 10 -i image%d.jpg animation.mp4
You obtain a movie file named animation.mp4.
Taking a picture by pressing a button
I mentioned earlier that one of the most interesting aspects of the use of PiCamera is that you can manage it in response to sensors and switches connected to GPIO pins. to get an idea of what The simplest example is to take a picture in response to the push of a button.
First you will need to connect a button to the board following the diagram shown below. So you will use two wire jumpers and a button by placing them on a breadboard.
Once you have prepared this simple circuit, open a file editor such as nano, and create a file where you will write the following code. First, as always, it imports the required modules
import time import picamera import Rpi.GPIO as GPIO
then set the GPIO pins, in particular the PIN 17, which will be set as input with the pull-up configuration.
GPIO.setmode(GPIO.BCM) GPIO.setup(17, GPIO.IN, GPIO.PUD_UP)
Finally, the last commands will activate the WebCam. When the pin will reveal a falling edge, caused by pressing the button directly connected to it, an image will be captured and saved as JPG.
camera = PiCamera() camera.start_preview() GPIO.wait_for_edge(17, GPIO.FALLING) camera.capture('/home/pi/image.jpg') camera.stop_preview()
Save the script in a file and run. An image.jpg file will be saved on your file system, containing the picture taken from the WebCam at the precise moment when you pressed the button.
Recording a video
But PiCamera can not only take pictures, but to record actual movies. So thanks to Python you can capture a video to be displayed later. This operation is very simple and does not differ much from the acquisition of simple images.
Open a new file named get_movie.py
$ nano get_movie.py
Here, too, you have to import the modules needed to use the PiCamera. Then define the PiCamera.
from picamera import PiCamera import time camera = PiCamera()
The following code is very simple and understandable.
camera.start_preview() camera.start_recording('/home/pi/video.h264') time.sleep(10) camera.stop_recording() camera.stop_preview()
Then execute the Python script
$ sudo python get_movie.py
After running this script, you will have a new video.h264 file containing the recorded video in 10 seconds. In order to view it, for example, you can use omxplayer application.
$ omxplayer video.h264
If you need to install omxplayer you have to enter the following command
$ sudo apt-get install omxplayer
If for example you want to view the video on a different operating system (Windows), you must convert the H.264 format into a more common format such as MP4. You can do this with avconv, launching the following command:
$ avconv -i video.h264 -c:v libx264 video.mp4
Or you can use another application called GPAC. It is very fast. Install it
$ sudo apt-get install -y gpac
and then launch the conversion with the MP4Box tool, included into this application.
$ MP4Box -fps 30 -add video.h264 video.mp4
Conclusions
This article is a brief overview about the use of PiCamera, a helpful accessory to be added to the Raspberry card. In future articles you will find out how to use the PiCamera to perform more complex operations by programming in Python. You will perform image analysis through the use of the OpenVC library.
Hi !
this page is really interesting thanks !
but id o have issue with following your steps…
when im trying to start a capture with GPIO and a button, sometimes my Pi is rebooting as soon as im pressing the boutton, or other time, the preview start but im stuck in preview and no picture or video ar recorded… do you have by chance any idea of what could be causing that ?
thanks you ๐
Maybe there will be some wrong with connection voltages with pressing button. Check the circuit and connection. Check the resistors. It is like Pi is turning down for protection.